Why I Chose to Build a Blog from Scratch for Klondike Solitaire
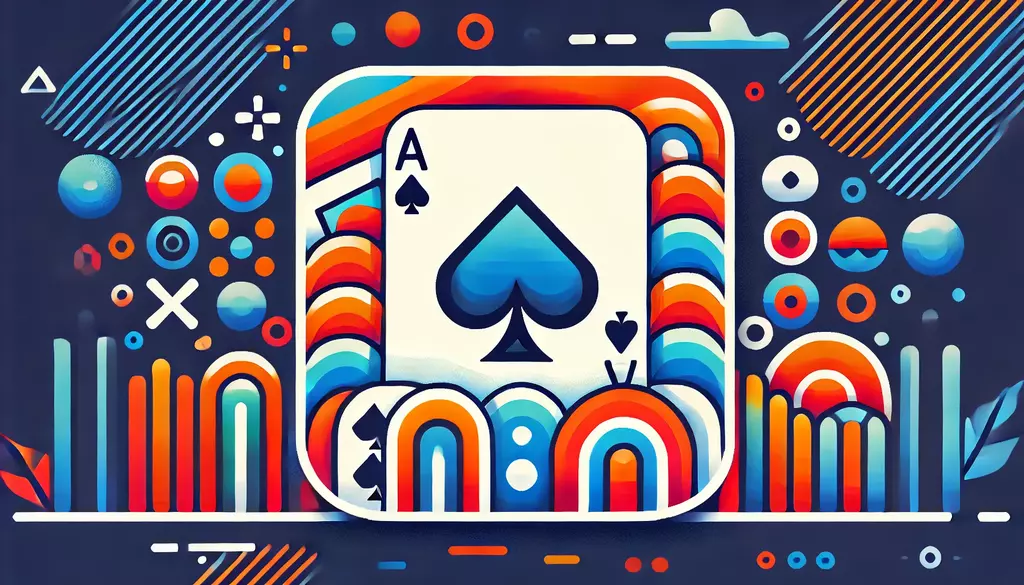
Building a blog from scratch for KlondikeSolitaire.com wasn’t the easiest route, but it was definitely the most rewarding. I had some clear goals in mind: I wanted it to be fast, lightweight, and tailored exactly to the site’s needs without the bloat of an off-the-shelf CMS. Going custom, I could avoid the extra features and overhead that can slow things down—things that just didn’t make sense for a simple, article-focused blog.
Why Skip the CMS?
Using a CMS like WordPress is tempting, with its themes, plugins, and quick setup. But as I dug deeper, I realized it came with too much baggage. I didn't want my site burdened with unnecessary code, features, or database queries. For Klondike Solitaire, where the blog is mainly static articles, a fully customized solution seemed like the better choice. So, I built exactly what I needed, fully optimized for performance and custom SEO elements, like a custom sitemap.
MVC: Keeping Things Organized
To keep the code clean and manageable, I relied on the Model-View-Controller (MVC) design pattern. This structure made a big difference in keeping everything organized:
- Model – Deals with data and logic, like pulling blog post data from Markdown files.
- View – Handles the display. I have a view for HTML pages and one for JSON responses, which is useful for future API-based features.
- Controller – Manages user requests, fetching data, and rendering content.
Here’s a snippet of the main BlogController
class:
class BlogController {
private $model;
private $view;
public function __construct(BlogModel $model, BlogViewInterface $view) {
$this->model = $model;
$this->view = $view;
}
public function handleRequest() {
$slug = $this->getSlug();
if ($slug === '') {
$this->renderHome();
} else {
$this->renderPost($slug);
}
}
private function renderHome() {
$posts = $this->model->getAllPosts();
$this->view->renderHome($posts);
}
}
It’s simple and clear—just what I need for a small blog without added complexities.
Building a Custom Sitemap Plugin
To help search engines find and index every page on the blog, I wrote a plugin to generate a custom XML sitemap. Here’s a quick look at the code:
class SitemapPlugin {
public function generateSitemap(array $posts): string {
$xml = new SimpleXMLElement('<urlset xmlns="http://www.sitemaps.org/schemas/sitemap/0.9"></urlset>');
foreach ($posts as $post) {
$url = $xml->addChild('url');
$url->addChild('loc', '/blog/' . $post['slug']);
$url->addChild('lastmod', $post['modified']);
$url->addChild('changefreq', 'monthly');
$url->addChild('priority', '0.8');
}
return $xml->asXML();
}
}
This plugin automates the sitemap updates, which helps keep the blog indexed correctly without extra effort on my part.
Markdown for Content
For simplicity, I store each blog post in a Markdown file. Each post starts with a bit of metadata (like title and tags), followed by the content. This keeps everything fast—no need for a CMS interface or database. Here’s an example:
---
title: "How to Win at Klondike Solitaire"
date: "2024-11-02"
tags: ["strategy", "gameplay"]
---
# How to Win at Klondike Solitaire
Winning at Klondike Solitaire requires patience, strategy, and a bit of luck...
Using Markdown means I can write and edit posts quickly and convert them to HTML without hassle.
Speed Benefits
Since each post is stored as a static file, the blog loads quickly without waiting on database queries. This structure makes for a smooth user experience and is ideal for content that doesn’t change often. For speed and simplicity, static files really are a win.
Adding Plugins and Flexibility
One of the goals was to keep the core structure lean but allow for flexibility. So, I added a basic plugin system that can handle features like the sitemap. For example:
if ($slug === 'sitemap.xml') {
$this->renderSitemap();
return;
}
With this setup, adding something like an RSS feed or another feature doesn’t mean rewriting core code. I can keep it clean and modular, and add only what’s needed.
Could I Make It Fully Static?
Yes! Though the blog is dynamic now, I could make it fully static by pre-generating each page. This would make hosting even simpler and even faster. No server-side code would be needed at all.
Final Thoughts
This project gave me a lot: a deep dive into the MVC structure, the beauty of Markdown, and a lightweight, high-performance blog that’s exactly what KlondikeSolitaire.com needed. It reaffirmed for me that sometimes, building from scratch can be worth the effort. And now, I have a solution that’s both flexible and minimal.
If you have specific needs and want total control, building your own blog is absolutely worth considering. It’s a refreshing way to keep things simple, speedy, and exactly how you want them.